hibernate配置文件
hibernate配置文件
hibernate.cfg.xml
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39
| <?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE hibernate-configuration PUBLIC "-//Hibernate/Hibernate Configuration DTD 3.0//EN" "http://www.hibernate.org/dtd/hibernate-configuration-3.0.dtd"> <hibernate-configuration> <session-factory> <property name="hibernate.connection.driver_class">com.mysql.jdbc.Driver</property> <property name="hibernate.connection.url">jdbc:mysql://localhost:3306/database</property> <property name="hibernate.connection.username">username</property> <property name="hibernate.connection.password">password</property>
<property name="hibernate.dialect">org.hibernate.dialect.MySQLDialect</property>
<property name="c3p0.min_size">5</property> <property name="c3p0.max_size">20</property> <property name="c3p0.timeout">1800</property> <property name="c3p0.max_statements">50</property>
<property name="hibernate.show_sql">true</property> <property name="hibernate.format_sql">true</property>
<property name="hibernate.hbm2ddl.auto">update</property>
<mapping resource="hbm/WFProcessProperty.hbm.xml" />
</session-factory> </hibernate-configuration>
|
映射关系文件
映射关系文件.hbm.xml
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22
| <?xml version="1.0" encoding="utf-8"?> <!DOCTYPE hibernate-mapping PUBLIC "-//Hibernate/Hibernate Mapping DTD 3.0//EN" "http://hibernate.sourceforge.net/hibernate-mapping-3.0.dtd">
<hibernate-mapping package="pojo"> <class name="Course" table="course"> <id name="id" type="long" column="id"> <generator class="assigned" /> </id> <property name="name" column="name" type="java.lang.String" not-null="false" /> <list name="students"> <key column="sid"></key> <list-index column="sid"></list-index> <many-to-many class="Student"></many-to-many> </list> </class> </hibernate-mapping>
|
hibernate-mapping标签的一些属性:
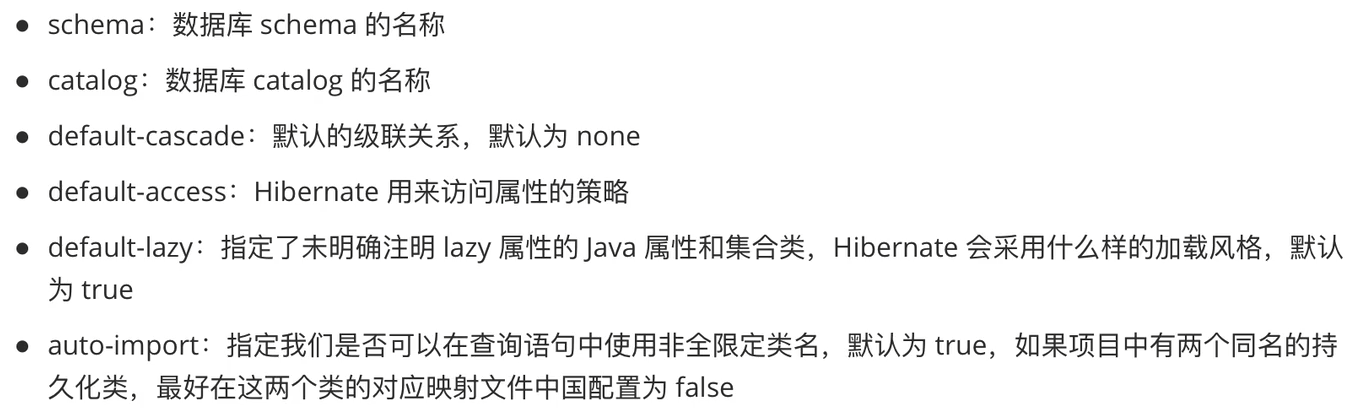
class标签的一些属性:
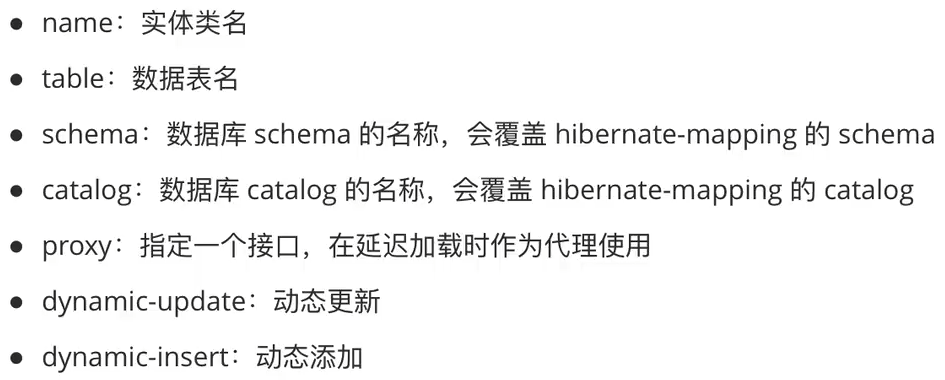
主键映射的方式:
- assigned:外部程序对id赋值
- native:由数据库对id赋值
- identity:采用数据库提供的主键生成机制
- increment:采用hibernate提供的主键生成机制
- sequence:采用数据库提供的sequence机制生成主键
- foreign:使用外部表的字段作为主键
property标签常用属性:
- update:字段是否可以修改,默认为true
- insert:字段是否可以添加,默认为true
- lazy:是否采用延迟加载策略,默认为true
集合类的常用属性:
- inverse:本表是否参与维护关系,默认为true,为false时会将维护权转让给对方类
- cascade:级联操作,当对本表操作时是否对集合中的类的对应表进行操作
测试类
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38
| public class CommonTest { private SessionFactory sessionFactory; private Session session; private Transaction transaction;
@Before public void init() { Configuration configure = new Configuration().configure(); sessionFactory = configure.buildSessionFactory(); session = sessionFactory.openSession(); transaction = session.beginTransaction(); }
@After public void destroy() { transaction.commit(); session.close(); sessionFactory.close(); }
@Test public void hibernateTest() { Student student = session.get(Student.class, 1L); System.out.println("修改前:"); System.out.println(student);
student.setName("李四"); session.update(student);
student = session.get(Student.class, 1L); System.out.println("修改后:"); System.out.println(student); } }
|
HQL
HQL:Hibernate Query Language,是一种面向对象的查询语言,可以完成查询、修改、删除操作,但不能完成插入操作
HQL是在Hibernate中作用的中间层语言,查询过程:java -> HQL -> SQL
hibernate使用小坑
无法检测到.hbm.xml文件
注意:这个文件和mybatis的mapper文件一样,如果需要放在java源码目录下,需要在pom.xml
中进行配置
1 2 3 4 5 6 7 8 9 10 11 12
| <build> <resources> <resource> <directory>src/main/java</directory> <includes> <include>**/*.properties</include> <include>**/*.xml</include> </includes> <filtering>true</filtering> </resource> </resources> </build>
|
输出对象导致stackoverflow
如果两个实体对象之间有循环依赖关系,如
1 2 3 4 5 6
| @Data public class Student { private long id; private String name; private List<Course> courses; }
|
1 2 3 4 5 6
| @Data public class Course { private long id; private String name; private List<Student> students; }
|
我们在使用lombok时可能不太注意,@Data注解为我们自动生成了toString()方法,Course类的toString()方法示例如下:
1 2 3 4 5 6 7
| public String toString() { return "Course{" + "id=" + id + ", name='" + name + '\'' + ", students=" + students + '}'; }
|
这里的返回拼接的students中又会去调用Student类的toString()方法,而Student类的toString()方法又会去调用Course类的toString()。。。进而出现无限的循环
解决办法:
手动实现任意一方的toString方法,取消对另一方的调用